
Bailey O.H.Embedded systems.Desktop integration.2005
.pdf
220 |
Chapter 7 / Hardware Development |
|
|
Combined, these devices offer a wide variety of options for our embedded system. We will not implement all of these devices at once, opting instead to develop the 1-Wire network interface and add the temperature sensor, real-time clock, and digital switch functions as needs require.
The Dallas 3-Wire Protocol
The 1-Wire protocol trades speed for distance. Before we continue, let’s briefly discuss the Dallas 3-Wire protocol. Our clock chip is the DS2404 and supports two methods of access. The first is the 1-Wire interface, which we examined above. The second method of access to this chip is the Dallas 3-Wire protocol. The benefit of the 3-Wire protocol is speed. Unlike the 1-Wire protocol, this protocol allows us to access the chip at much higher speeds. The three wires are defined as:
Reset line
Clock line
Data line
Using the 3-Wire protocol, the reset line is used to turn communication on and off. If the reset line is low, then no 3-Wire communications can take place. If the reset line is high, the next step in communicating is to place the clock in the proper state and send the data bytes. Once the data has been sent, the clock line is changed back to an idle state. What makes this dual interface interesting is that in addition to the time, the DS2404 also has memory and both the 1- and 3-Wire interfaces can be used so long as you can handle contention issues. This means that the 1-Wire could be used for exchanging data over long distances with a master while the 3-Wire is used to communicate with a local processor that collects and stores data. Before the 3-Wire protocol can be used, the 1-Wire interface must be initialized and reset.

Chapter 7 / Hardware Development |
221 |
|
|
A Light-Duty 1-Wire Network
There are several different ways to implement the 1-Wire protocol. Which method you use depends on the overall distance you need the network to operate within. Let’s first explore a light-duty 1-Wire interface. The Dallas documentation defines a light-duty interface as one that operates at distances no longer than 3 meters. Now you may be asking why anyone would want to implement a 3-meter interface, so here is one example of where this would work very well. If you had a walk-in freezer control accessible on the outside of the freezer, you could attach the sensor inside the freezer by simply drilling a hole to the inside area. You could also keep track of a room’s temperature by having multiple sensors on different walls. In either of these situations, a light-duty 1-Wire interface would work just fine and is inexpensive to implement.
This interface requires only one resistor (2.2 kOhm resistor (Radio Shack 271-1121)), a +5-volt power supply, and a ground wire. While we are using the BASIC Stamp for developing this interface, it can just as easily be implemented with the PIC or PSoC.
+5V
2.2 kOhm
Data
GND
Device |
Device |
Device |
1 |
2 |
3 |
Figure 7-17
Chapter 7

222 |
Chapter 7 / Hardware Development |
|
|
As you can see from the figure, only three wires are needed to build this interface and only one processor pin is used. Implementing this circuit takes only a few minutes and a 2.2 kOhm resistor. In Figure 7-18 the circuit has been implemented on a solderless breadboard.
Figure 7-18
The power pin of the DS1822 is always located on the left with the flat side of the device facing up. In Figure 7-19 you can see how few components are in this circuit and how easy it is to implement.
Figure 7-19
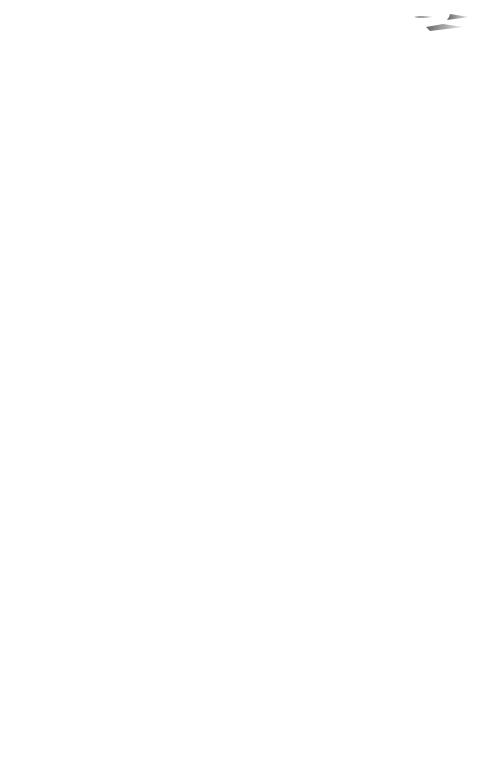
Chapter 7 / Hardware Development |
223 |
|
|
The schematic in Figure 7-20 illustrates how our completed circuit will be assembled. Since this board does not have its own power supply, both power and ground must be provided from an external source. Since the 1-Wire interface requires +5 volts and ground, +5 is supplied through pin 1 on either JP8 or JP1, and ground is provided on pin 3 of the same connectors. Pin 2 of JP8 and JP1 is connected to the 1-Wire data line of the DS1822. To provide better 1-Wire communications, R4 is a 2.2 K resistor between +5 volts and the 1-Wire data line.
Chapter 7
Figure 7-20
The DS2404 has both 1-Wire and 3-Wire I/O. Headers JP8 and JP1 are the 1-Wire interface, while headers JP2 and JP3 interface to a 3-Wire bus. Jumper JP4 is the interrupt output. The jumper marked 1KHZ is actually a 1-kHz output and is strictly for test purposes. BAT1 is the CR2032 holder for battery backup.
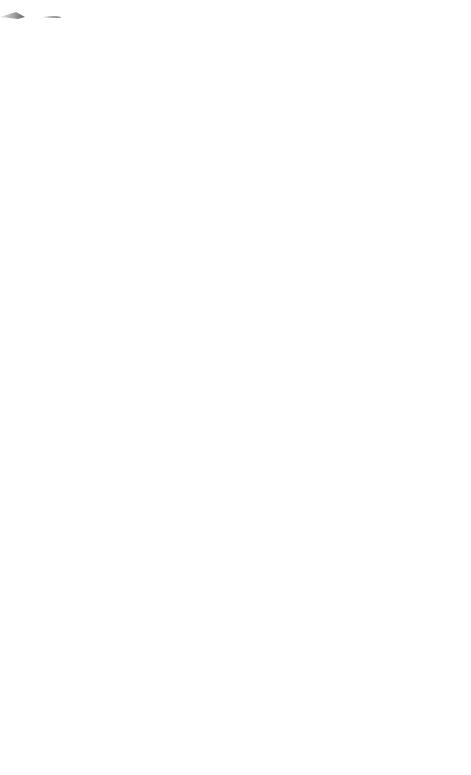
224 |
Chapter 7 / Hardware Development |
|
|
A Simple 1-Wire Test Program
To test this circuit, start the BASIC Stamp Editor and type in the following program:
Listing 7-2
//{$STAMP BS2p}
//{$PBASIC 2.5}
//Test Program for Dallas 1-Wire Thermometer
//for Embedded Systems Desktop Integration
//Copyright 2004 - Oliver H. Bailey
//
// This program uses PIN 15 for both Input and Output
// Pin 15 - Transmit/Receive 1-Wire Data |
<=> |
// |
|
Temp VAR Word |
// Temperature Storage Variable |
TempLo VAR Temp.LOWBYTE |
// Temperature Low Order Byte |
TempHi VAR Temp.HIGHBYTE |
// Temperature High Order Byte |
TempSign VAR temp.BIT11 |
// Temperature Sign Bit |
signBit VAR Bit |
|
tempCel VAR Word |
// Centigrade |
tempFar VAR Word |
// Fahrenheit |
ChkLoop VAR Byte |
// Three attempts |
//This is a very simple program for testing a DS1822 Temp sensor. Rather than using
//the traditional Read ROM command we simply make an attempt to read the temperature.
//If a device is present then the temperature is returned; otherwise it is assumed
//no DS1822 is attached.
ChkLoop = |
0 |
// Set to Zero Tries |
Main: |
|
|
GOSUB ChkDev |
// Check for DS1822 |
|
// Reaching this line requires a response from the DS1822 |
||
tmpLoop: |
// Read temperature loop start |
|
GOSUB Read_Temp |
// Gosub read temperature |
|
DEBUG HOME, SDEC tempCel, " C", CR |
// Display Centigrade |
|
DEBUG SDEC tempFar, " F", CR |
// Display Fahrenheit |
|
PAUSE 500 |
// Wait |
|
GOTO tmpLoop |
// Start again |
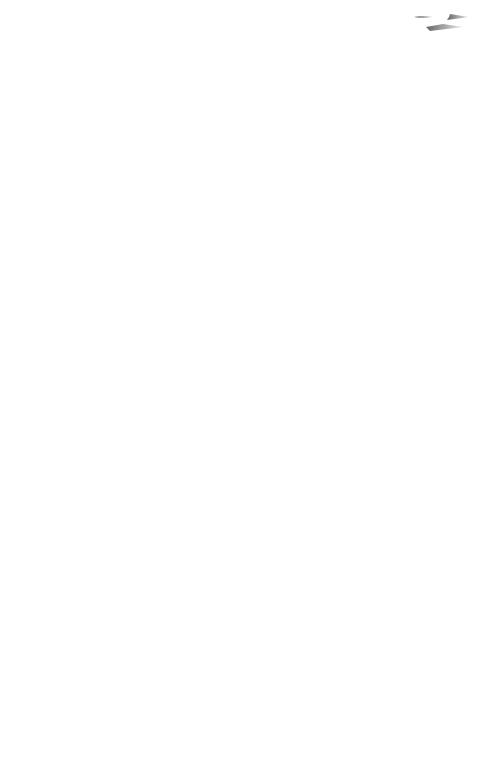
|
Chapter 7 / Hardware Development |
225 |
|
|
|
|
|
// If the code reaches here, it was by mistake. |
|
|
|
GOTO forever |
// Loop forever |
|
|
// Check Device Subroutine |
|
|
|
ChkDev: |
|
|
|
OWOUT 15, 1, [$CC, $44] |
// Check for temperature |
|
|
NotDone: |
|
|
|
PAUSE 25 |
// Wait for response |
|
|
OWIN 15, 4, [Temp] |
// Check Status |
|
|
ChkLoop = ChkLoop+1 |
// Increment Loop Counter |
|
|
IF ChkLoop = 100 THEN no_Dev |
// Max Tries then Stop |
|
|
IF Temp = 0 THEN |
// No Response |
|
|
GOTO NotDone |
// Otherwise Try Again |
|
|
ENDIF |
|
|
|
RETURN |
// Return if Found |
|
|
// Read and Convert Temperature |
|
|
|
Read_Temp: |
|
|
|
OWOUT 15, 1, [$CC, $44] |
// Check for temperature |
|
|
notReady: |
// Start completion loop |
|
|
PAUSE 25 |
// Wait for response |
|
|
OWIN 15, 4, [Temp] |
// Check results |
|
|
IF Temp = 0 THEN notReady |
// Not done, start again |
|
|
OWOUT 15, 1, [$CC, $BE] |
// Read Scratchpad Temp |
|
|
OWIN 15, 2, [TempLo, TempHi] |
// Get Temp Bytes |
|
|
signBit = TempSign |
// Get sign bit (0 = +, 1 = -) |
|
|
tempCel = Temp |
// Store to variable |
|
|
tempCel = tempCel >> 4 |
// Store Temp to variable |
|
|
IF (signBit = 0) THEN PosCel |
// Shift to get actual temperature |
|
|
tempCel = tempCel | $FF00 |
// Check for sub-zero |
|
|
|
// Adjust if below zero |
|
|
PosCel: |
|
|
|
tempFar = tempCel */ $01CD |
// Positive Temperature |
|
|
IF signBit = 0 THEN PosFar |
// Adjust for display |
|
|
tempFar = tempFar | $FF00 |
// Check for sub-zero |
|
|
|
// Adjust Fahrenheit scale |
|
|
PosFar: |
|
|
|
tempFar = tempFar + 32 |
// Not sub-zero |
|
|
RETURN |
// Add 32 for Fahrenheit display |
|
|
|
// All Done, return |
|
Chapter 7
no_Dev:

226 |
|
Chapter 7 / Hardware Development |
|
|
|
|
|
|
|
|
|
|
DEBUG "No DS1822 Device Found", CR |
// No response at startup so display |
|
|
|
|
// message |
forever: |
// |
||
|
GOTO forever |
// and loop forever |
This code listing performs the following functions:
1.Tests for the existence of a DS1822.
2.Runs in a continuous loop, taking a new temperature reading every 500 milliseconds. This value could be lengthened but at half a second any temperature changes will be displayed quickly.
3.A safety “loop forever” routine if something goes wrong.
That’s all the code needed to check out our circuit. If it works, you will see a screen similar to the one in Figure 7-21.
Figure 7-21
This is a very simple test. The first line displays the temperature in Celsius and the second line displays the temperature in Fahrenheit. This procedure confirms that our circuit works just fine. While we haven’t added the DS2404 yet, we now know the circuit will read the DS1822 temperature chip. Next, we’ll add the DS2404 time and date chip before building the main I/O board.

Chapter 7 / Hardware Development |
227 |
|
|
Adding the DS2404
Your circuit would be complete if you only wanted to watch the temperature rise or fall, but we need to add support for the date and time. The temperature sensor looks like a transistor; this is called a TO92 case. It is round with a flat spot on the face of the part that has the part number inscribed on it. Mounting this type of component is very easy and the holes can be close together (0.1"). The DS2404, on the other hand, does not come in the same style case. The DS2404 has three packaging options but for our purpose we will use the standard 16-pin dual inline package (DIP). This will make handling the chip easier and prevent us from having to worry about surface mount parts. The DS2404 is a very versatile but complicated part. The following list shows the major features of this device:
1-Wire interface
3-Wire interface
Reset input
Timer interrupt
1 Hz output
ROM serial number
512-byte battery-backed RAM
Time and date counters
Power cycle counter
External battery backup
There are two voltage connections for power and battery backup connections to keep the clock running if power goes down. Figure 7-22 shows the DS2404 16-pin DIP layout.
Chapter 7

228 |
Chapter 7 / Hardware Development |
|
|
VCC 2.8 - 5.5 Volts |
1 |
DS2404-001 |
16 VCC 2.8 - 5.5 Volts |
|
|
|
|
|
|
Interrupt Output |
2 |
|
15 |
Crystal Connection |
3-Wire Reset |
3 |
|
14 |
Crystal Connection |
3-Wire Data |
4 |
|
13 |
Ground |
1-Wire Data |
5 |
|
12 |
Not Connected |
3-Wire Data Clock |
6 |
|
11 |
1 Hz Output |
Not Connected |
7 |
|
10 |
Battery Backup |
Ground |
8 |
|
9 |
Battery Backup |
Figure 7-22
Of all the 1-Wire products this device is one of the most complicated. Since this is a book about communications, we will implement both the 1- and 3-Wire interfaces even though we are interested only in the timekeeping, battery backup, power cycle counter, and memory functions. The main reason we’ve selected this device is for the 1-Wire network access. This device can be added to our existing 1-Wire network with no additional usage of microcontroller pins, but utilizing the features of this chip will require some software development. For this chapter, however, we only want to build and test the circuit to be certain the device is working and the circuit design is sound. Figure 7-23 illustrates the 1-Wire side of the DS2404.
+5 Volts
|
+5 Volts |
|
-001 |
1-Wire |
S2404D |
Data |
|
Network |
|
|
Gnd |
32768 Hz
Clock Crystal
2.8 - 5.5 Volts
Gnd
Figure 7-23

Chapter 7 / Hardware Development |
229 |
|
|
The only components required to make this circuit work is a clock crystal and battery (CR2032 type). Our test circuit will look similar to Figure 7-24.
Chapter 7
Figure 7-24
Note:
This chip has a built-in cycle counter. The battery-backed functions of this chip work across a pretty wide voltage range. This means you could set a voltage filtering system that would report voltage drops based on the battery value. This could be used to track brownouts or power surges.
Test Software for the DS2404
Now it’s time to see if our circuit works. Testing the device consists of detecting the presence of the device, writing a few bytes of data to the device’s scratchpad memory, and then reading the data back. This will prove the device has power and the 1-Wire network is working properly. The following program will accomplish this task.